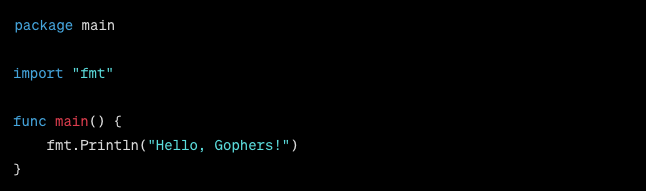
Introduction
Welcome to your first steps in the fascinating world of Go programming! Go, colloquially known as Golang, is an open-source programming language developed at Google. It's designed to be simple yet powerful, offering efficiency akin to lower-level languages like C++, combined with the ease of use of higher-level ones such as Python or JavaScript.
The standout features of Go include its efficiency in processing, scalability, and powerful concurrency handling capabilities. These traits make it an increasingly popular choice for developing web servers, data pipelines, and even for tasks in data science!
Installation & Setup
First things first, we need to install Go on our machines. Here's how you can do it based on your operating system:
- Windows: Download the MSI installer from the official Go downloads page and run it.
- macOS: You can use the same download page, or for a faster route, use Homebrew by typing
brew install go
in your terminal. - Linux: The download page also has a tarball for Linux. Or you can use your distribution's package manager, like
sudo apt-get install go
for Ubuntu.
Next, choose a text editor or Integrated Development Environment (IDE). Go is supported by many popular editors like VS Code, Atom, and GoLand. We'll use Visual Studio Code for our examples since it's free and has great Go support with the Go extension.
The Structure of a Go Program
Now that we've got our toolbox ready let's understand the blueprint of a basic Go program. In Go, every application starts running in a package called main
. Inside this package, the function named main
is the entry point of our program. All this code usually lives in a file named main.go
. Here's what it looks like:
package main
import "fmt"
func main() {
fmt.Println("Hello, Gophers!")
}
This program simply prints "Hello, Gophers!" to the console. fmt
is a package that's included in the Go standard library, which brings us to our next topic.
Basic Concepts in Golang
Before we dive deeper, let's familiarize ourselves with some basic concepts:
- Variables: You can declare a variable using the
var
keyword, or use:=
for shorthand declaration and initialization. For example:
var str string
str = "Hello, Gophers!"
// Or shorthand
str := "Hello, Gophers!"
- Data types: Go has various data types including
string
,int
,float64
,bool
, and more. - Operators: Go uses common arithmetic operators (
+
,-
,*
,/
,%
), assignment operators (=
,+=
,-=
, etc.), comparison operators (==
,!=
,<
,>
, etc.), and logical operators (&&
,||
,!
). - Control Structures: Go has
if
,else
,switch
,for
, andrange
for flow control. - Functions: You can declare a function with the
func
keyword. Functions can take zero or more arguments and can also return a value.
Packages and Imports
In Go, code is organized into packages. A package is a collection of source files in the same directory that are compiled together. For example, fmt
is a package that we imported for printing.
We import packages using the import
keyword, and Go has a vast standard library of packages like fmt
, math
, net/http
, and many more.
import (
"fmt"
"math"
)
func main() {
fmt.Println(math.Sqrt(16)) // prints "4"
}
This program uses the math
package to compute the square root of 16. Besides standard packages, you can create your own or use ones shared by the community.
By understanding these concepts, you are well on your way to mastering Go! In the next part, we'll discuss Go tools, working with multiple files, and more. Stay tuned!
Watch the youtube video above to dive deeper into the various components of a go program.
Happy Coding, Gophers!